Exploring Core Android Components: Activities, Services, Broadcast Receivers, and Content Providers
Android, as an operating system, provides developers with a set of core building blocks to create responsive, secure, and feature-rich applications. These building blocks, known as components, work together to deliver a cohesive user experience. Understanding these components is critical for Android app development. In this blog, we'll dive deep into Android’s primary components:
- Activities
- Services
- Broadcast Receivers
- Content Providers
- Android Manifest and Permissions
1. Activities
What is an Activity?
An Activity represents a single screen in an Android app. It is a crucial part of any Android application because each interaction with the user typically happens through an Activity. For example, in a simple note-taking app, one Activity might display a list of notes, and another could allow you to create a new note.
Key Concepts of Activities:
- Navigation: Activities work with other components such as
Fragments
orIntents
to handle screen navigation. You can start new Activities, pass data between them, and manage back stack navigation usingIntent
. - Layouts: Activities are linked to user interface layouts defined in XML files. These layouts contain Views and ViewGroups that make up the visual representation of an Activity.
Lifecycle: Activities have a well-defined lifecycle controlled by a series of callback methods such as onCreate()
, onStart()
, onResume()
, onPause()
, onStop()
, and onDestroy()
. These methods ensure that activities respond properly to user interactions and system events.
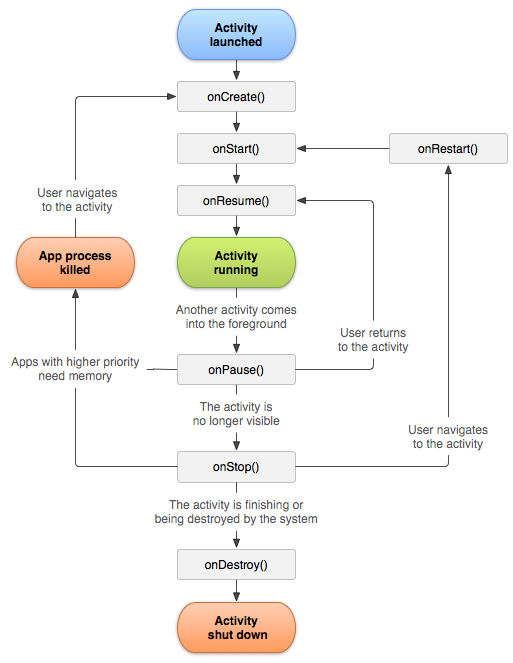
Example of Activity:
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
}
2. Services
What is a Service?
A Service is a component that performs long-running background tasks without interacting directly with the user. Services continue to operate even if the user switches to another app or when the app is not in the foreground.
Key Types of Services:
- Started Services: These are initiated by calling
startService()
. Once started, a service can run indefinitely, performing tasks like downloading a file or playing music. - Bound Services: These allow a component (like an Activity) to bind to a service and interact with it. This is useful when the service needs to provide a communication channel, like a Messenger or an AIDL interface.
Example of Service:
public class MyService extends Service {
@Override
public int onStartCommand(Intent intent, int flags, int startId) {
// Perform background task here
return START_STICKY;
}
@Override
public IBinder onBind(Intent intent) {
return null; // Return binder for bound services
}
}
3. Broadcast Receivers
What is a Broadcast Receiver?
A Broadcast Receiver is a component that allows an application to respond to broadcast messages from other applications or from the system itself. These broadcasts can be events like a system boot, network state change, or a low battery warning.
Types of Broadcasts:
- System Broadcasts: These are sent by the Android system for various system-level events. For instance,
ACTION_BOOT_COMPLETED
is triggered when the device finishes booting. - Custom Broadcasts: Applications can also send custom broadcasts. This is often used for internal application communication between different parts of the app.
Example of Broadcast Receiver:
public class MyReceiver extends BroadcastReceiver {
@Override
public void onReceive(Context context, Intent intent) {
if (intent.getAction().equals(Intent.ACTION_BOOT_COMPLETED)) {
// Code to execute when the system boots up
}
}
}
4. Content Providers
What is a Content Provider?
A Content Provider is responsible for managing access to structured data, such as files, databases, or even data on a different application. Content Providers act as a standard interface for sharing data between different applications securely.
Common Use Cases:
- Sharing contacts information with other apps.
- Accessing media (like images or audio files) from another app.
Content Providers use URIs to query and manipulate data. The system's ContentResolver object handles interactions with a Content Provider.
Example of Content Provider Usage:
Cursor cursor = getContentResolver().query(
ContactsContract.Contacts.CONTENT_URI,
null, null, null, null);
while (cursor.moveToNext()) {
String name = cursor.getString(cursor.getColumnIndex(ContactsContract.Contacts.DISPLAY_NAME));
// Use the contact name
}
5. Android Manifest and Permissions
What is the Android Manifest?
The Android Manifest is an essential XML file in every Android app that declares the app’s components, permissions, and hardware features. It provides essential information for the Android system to manage the app.
Some key elements declared in the AndroidManifest.xml
:
- Activities, Services, Broadcast Receivers, and Content Providers
- Permissions: This section defines the permissions required by your app, such as access to the internet, camera, location, etc.
Example of Android Manifest:
<uses-permission android:name="android.permission.INTERNET"/>
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<service android:name=".MyService"/>
<receiver android:name=".MyReceiver">
<intent-filter>
<action android:name="android.intent.action.BOOT_COMPLETED"/>
</intent-filter>
</receiver>
<provider
android:name=".MyContentProvider"
android:authorities="com.example.myapp.provider"
android:exported="false" />
Permissions in Android:
Permissions are used to secure access to sensitive data and hardware components like the camera, location services, or phone state. Android categorizes permissions into two types:
- Normal Permissions: Automatically granted by the system. These include permissions like accessing the internet or setting alarms.
- Dangerous Permissions: These require explicit user consent at runtime (from Android 6.0 and above). Examples include accessing the user's contacts, location, or storage.
Requesting Dangerous Permissions at Runtime:
if (ContextCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION)
!= PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(this,
new String[]{Manifest.permission.ACCESS_FINE_LOCATION},
MY_PERMISSIONS_REQUEST_LOCATION);
}
Conclusion
Understanding Android’s core components — Activities, Services, Broadcast Receivers, and Content Providers — is essential to building functional and robust Android applications. These components, combined with proper use of the Android Manifest and permissions, provide the foundation for managing app behavior, interacting with the system, and maintaining security and user privacy.
Each component plays a distinct role in how the app functions, handles background tasks, interacts with the system, or shares data with other applications. Mastering these will help you build more efficient, secure, and user-friendly Android applications.
Comments ()